We’re trusted by 100,000+ Australian businesses
“First class support”
“Easy to use!”
“Couldn’t be happier!“
“Surprisingly sophisticated“
“Great customer service!”
“Very easy & effective accounting software!“
“The level of service & care was extraordinary“
“Great professional user friendly software and support“
“Super clean & simple product”
“Best software on the market!”
“Great package on all levels”
“Speak to real life support people”
“Great product, even greater service!“
“Far more affordable than the competitors”
“It has saved me so much time!”
Industry recognition and reviews
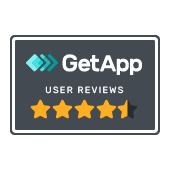
GetApp
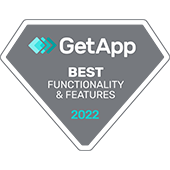
GetApp

Software Advice
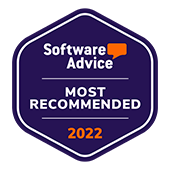
Software Advcie
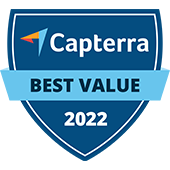
Capterra
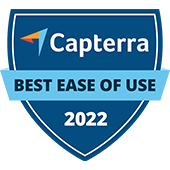
Capterra
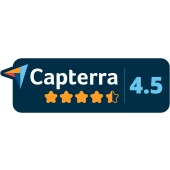
Capterra
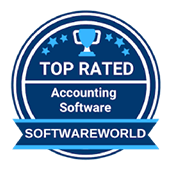
Software World
Here’s what our customers have to say
Reckon One has helped thousands of businesses gain a clearer picture of their cash flow and helped them save hours on invoicing and accounting admin.
Read their stories and see how Reckon One can help your business achieve success.
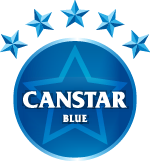
Canstar Blue’s Reckon One review
Canstar Blue answers your much-anticipated question – should you choose Reckon accounting software for your business? They dive deep into the features and benefits of Reckon One for businesses – highlighting our affordability against other competitors.
World Class Support
It’s not just the fancy software, it’s about the friendly face
Our support alone is a strong reason to choose Reckon One. We’re always here to help. Unlike many of our competitors, you can phone us directly to receive immediate help. We also offer a call-back service, email support and have a 24/7 online community that is free to join!
Try Reckon One free for 30 days
Cancel anytime.